Radix Combobox
Live example
Components
Explore the Ariakit components used in this example:
Basic structure
<RadixPopover.Root>
<RadixPopover.Anchor asChild>
</RadixPopover.Anchor>
<RadixPopover.Content asChild>
</RadixPopover.Content>
</RadixPopover.Root>
Composing PopoverContent
with ComboboxList
Rather than using the ComboboxPopover
component from Ariakit, we can use the Radix PopoverContent
in conjunction with the Ariakit ComboboxList
component, which doesn't bundle popover features.
Here are a few things to keep in mind when assembling these components:
- 1
A combobox typically doesn't auto-focus when it opens, so we need to stop the Radix
onOpenAutoFocus
event from performing its default behavior:<RadixPopover.ContentonOpenAutoFocus={(event) => event.preventDefault()}> - 2
We need to stop Radix from closing the popover when interacting with the
Combobox
or any component withinComboboxList
:<RadixPopover.ContentonInteractOutside={(event) => {const isCombobox = comboboxElement === event.target;const inListbox = listboxElement?.contains(event.target);if (isCombobox || inListbox) {event.preventDefault();}}}> - 3
Finally, we must explicitly set
role="listbox"
on theComboboxList
component, otherwise Radix will overwrite it withrole="dialog"
.
More examples
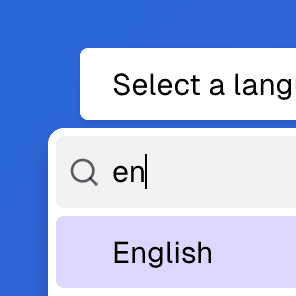
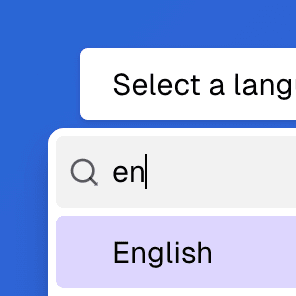
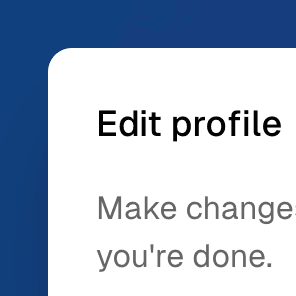
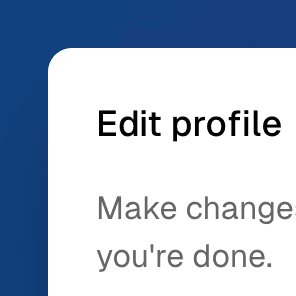
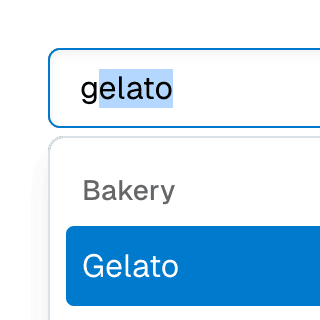
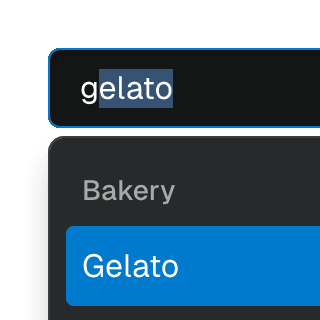
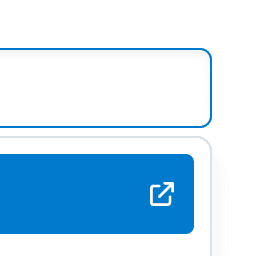
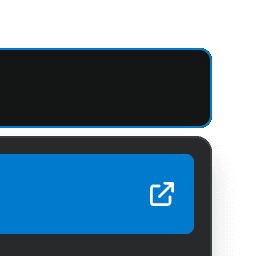
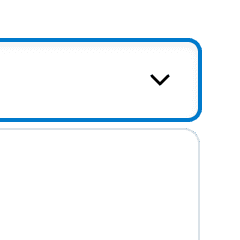
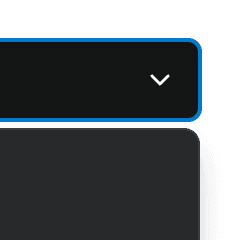
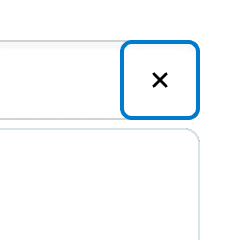
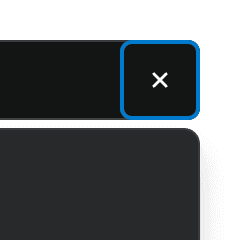
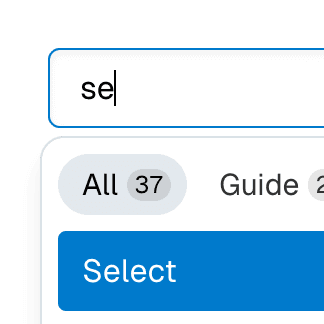
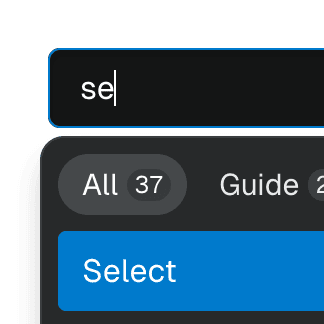
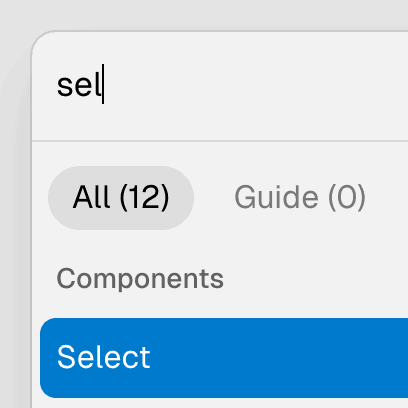
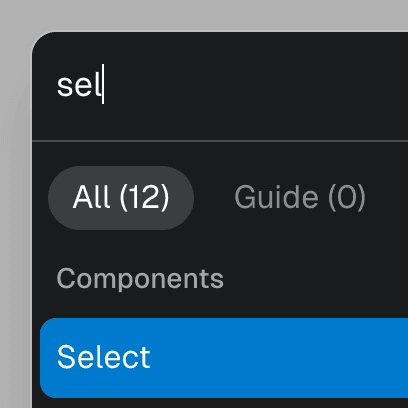