Multi-selectable Combobox
Allowing Combobox to select multiple options by passing an array value to the selectedValue
prop.
Apple
Bacon
Banana
Broccoli
Burger
Cake
Candy
Carrot
Cherry
Chocolate
Cookie
Cucumber
Donut
Fish
Fries
Grape
Green apple
Hot dog
Ice cream
Kiwi
Lemon
Lollipop
Onion
Orange
Pasta
Pineapple
Pizza
Potato
Salad
Sandwich
Steak
Strawberry
Tomato
Watermelon
1234567891011121314151617181920212223242526272829303132333435363738394041424344454647484950515253
import * as Ariakit from "@ariakit/react";import { matchSorter } from "match-sorter";import { useMemo, useState, useTransition } from "react";import list from "./list.ts";import "./style.css";export default function Example() {const [isPending, startTransition] = useTransition();const [searchValue, setSearchValue] = useState("");const [selectedValues, setSelectedValues] = useState(["Bacon"]);const matches = useMemo(() => matchSorter(list, searchValue), [searchValue]);return (startTransition(() => {setSearchValue(value);});}}>Your favorite foodplaceholder="e.g., Apple, Burger"className="combobox"/>className="popover"aria-busy={isPending}>{matches.map((value) => (key={value}className="combobox-item">{value}))}{!matches.length && <div className="no-results">No results found</div>});}import * as Ariakit from "@ariakit/react";import { matchSorter } from "match-sorter";import { useMemo, useState, useTransition } from "react";import list from "./list.ts";import "./style.css";export default function Example() {const [isPending, startTransition] = useTransition();const [searchValue, setSearchValue] = useState("");const [selectedValues, setSelectedValues] = useState(["Bacon"]);const matches = useMemo(() => matchSorter(list, searchValue), [searchValue]);return (startTransition(() => {setSearchValue(value);});}}>Your favorite foodplaceholder="e.g., Apple, Burger"className="combobox"/>className="popover"aria-busy={isPending}>{matches.map((value) => (key={value}className="combobox-item">{value}))}{!matches.length && <div className="no-results">No results found</div>});}
Components
Related examples
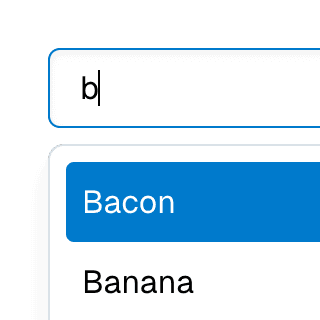
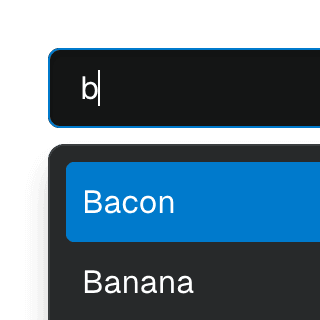
Combobox filteringListing suggestions in a Combobox component based on the input value using React.startTransition to ensure the UI remains responsive during typing.
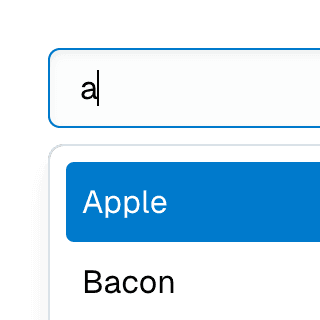
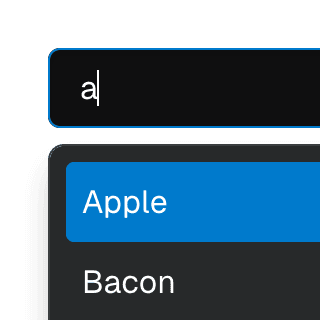
Combobox with integrated filterFiltering options in a Combobox component through an abstracted implementation using React.useDeferredValue, resulting in a simple higher-level API.
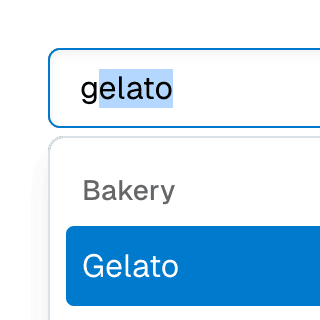
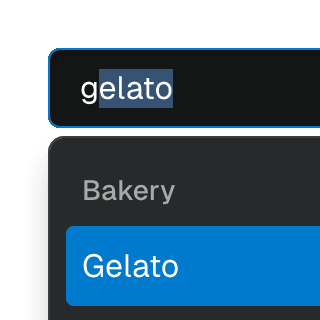
ComboboxGroupOrganizing Combobox items into labelled groups using the ComboboxGroup and ComboboxGroupLabel components in React.
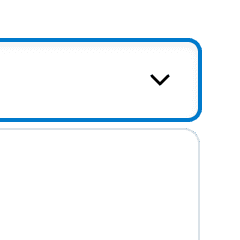
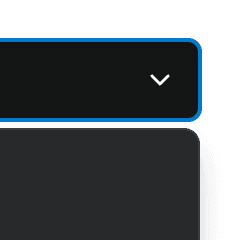
ComboboxDisclosureOpening and closing a Combobox with the help of a button rendered next to it using the ComboboxDisclosure component.
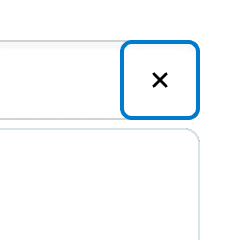
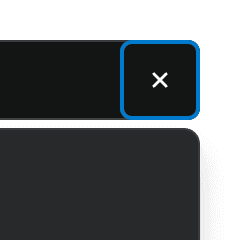
ComboboxCancelReseting the value of a Combobox with a button rendered next to it using the ComboboxCancel component.
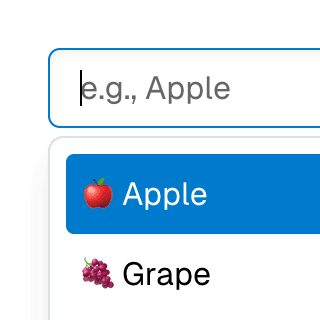
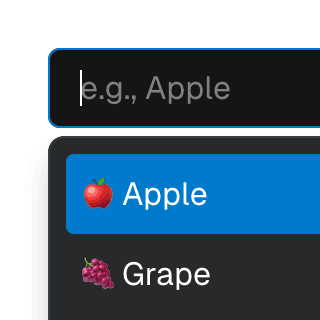
Animated ComboboxAnimating a Combobox using CSS transitions in React. The component waits for the transition to finish before completely hiding the popover.
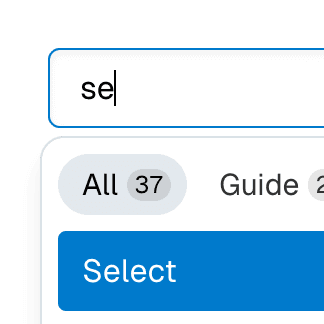
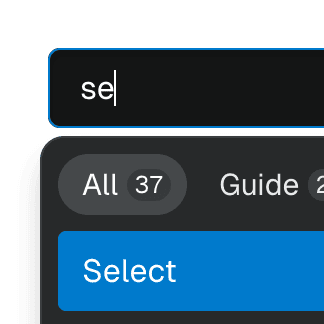
Combobox with TabsOrganizing Combobox with Tab components that support mouse, keyboard, and screen reader interactions. The UI remains responsive by using React.startTransition.
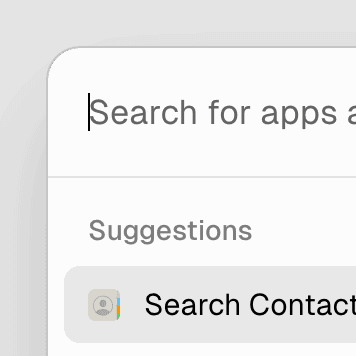
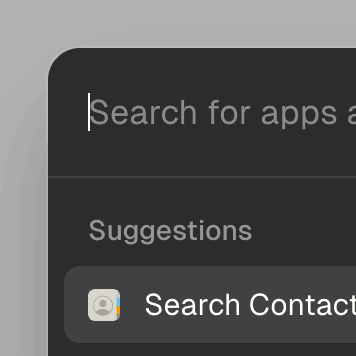
Command MenuCombining Dialog and Combobox to enable users to search a command list in a Raycast-style modal.