Select with Combobox
Combining Select and Combobox to create a dropdown with a search field that can be used to filter items.
Favorite fruit
Apple
Bacon
Banana
Broccoli
Burger
Cake
Candy
Carrot
Cherry
Chocolate
Cookie
Cucumber
Donut
Fish
Fries
Grape
Green apple
Hot dog
Ice cream
Kiwi
Lemon
Lollipop
Onion
Orange
Pasta
Pineapple
Pizza
Potato
Salad
Sandwich
Steak
Strawberry
Tomato
Watermelon
1234567891011121314151617181920212223242526272829303132333435363738394041424344454647484950515253
import * as Ariakit from "@ariakit/react";import { matchSorter } from "match-sorter";import { startTransition, useMemo, useState } from "react";import list from "./list.ts";import "./style.css";export default function Example() {const [searchValue, setSearchValue] = useState("");const matches = useMemo(() => {return matchSorter(list, searchValue, {baseSort: (a, b) => (a.index < b.index ? -1 : 1),});}, [searchValue]);return (<div className="wrapper">startTransition(() => {setSearchValue(value);});}}><div className="combobox-wrapper">placeholder="Search..."className="combobox"/></div>{matches.map((value) => (key={value}className="select-item"/>))}</div>);}import * as Ariakit from "@ariakit/react";import { matchSorter } from "match-sorter";import { startTransition, useMemo, useState } from "react";import list from "./list.ts";import "./style.css";export default function Example() {const [searchValue, setSearchValue] = useState("");const matches = useMemo(() => {return matchSorter(list, searchValue, {baseSort: (a, b) => (a.index < b.index ? -1 : 1),});}, [searchValue]);return (<div className="wrapper">startTransition(() => {setSearchValue(value);});}}><div className="combobox-wrapper">placeholder="Search..."className="combobox"/></div>{matches.map((value) => (key={value}className="select-item"/>))}</div>);}
Related examples
Select with Combobox and TabsAbstracting Select to work alongside Combobox and Tab components, presenting a searchable, tabbed dropdown.
Menu with ComboboxCombining Menu and Combobox to create a dropdown menu with a search field that can be used to filter menu items.
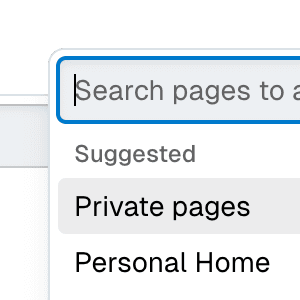
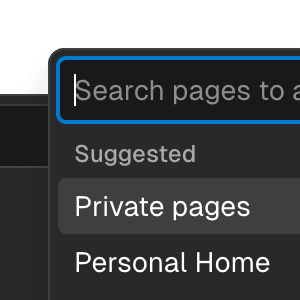
Submenu with ComboboxNesting Notion-style dropdown menus with search & autocomplete features by combining Menu with Combobox.
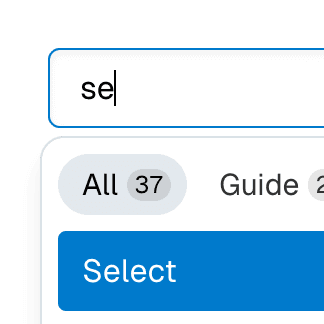
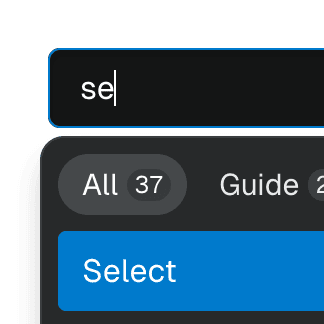
Combobox with TabsOrganizing Combobox with Tab components that support mouse, keyboard, and screen reader interactions. The UI remains responsive by using React.startTransition.
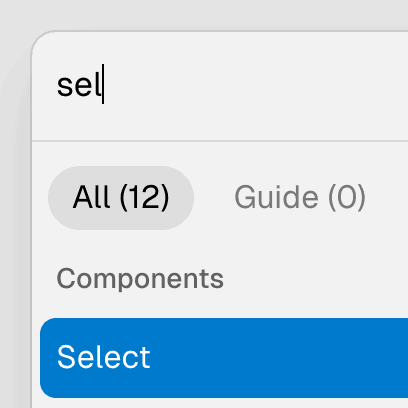
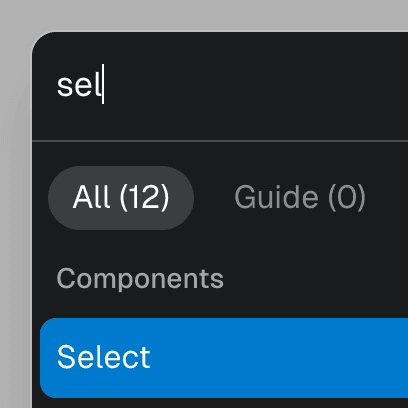
Command Menu with TabsCombining Dialog, Tab, and Combobox from Ariakit React to build a command palette component.
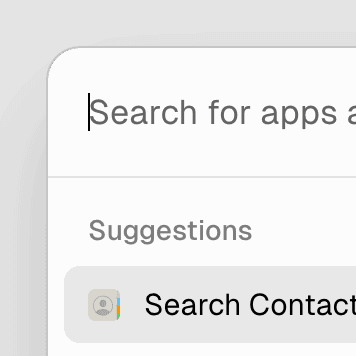
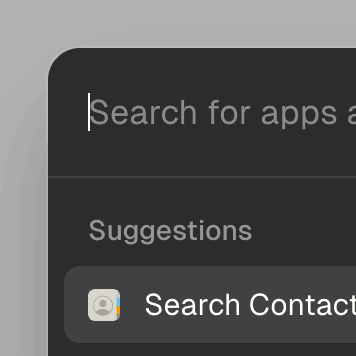
Command MenuCombining Dialog and Combobox to enable users to search a command list in a Raycast-style modal.