Radix Select with Combobox
Components
Explore the Ariakit components used in this example:
Basic structure
<RadixSelect.Root>
<RadixSelect.Trigger />
<RadixSelect.Content>
<RadixSelect.Item asChild>
</RadixSelect.Item>
</RadixSelect.Content>
</RadixSelect.Root>
Filtering options
The Ariakit Combobox component doesn't dictate how you filter the items. It focuses solely on the ComboboxItem
elements you render. Consequently, you can render items conditionally based on the value
state.
We use the setValue
callback in combination with React.startTransition
to update our search value state without blocking the UI:
const [searchValue, setSearchValue] = useState("");
React.startTransition(() => {
setSearchValue(value);
});
}}
>
You're free to use any matching algorithm or library to filter the items. In this example, we use match-sorter:
const matches = useMemo(() => {
return matchSorter(languages, searchValue, {
keys: ["label", "value"],
});
}, [languages, searchValue]);
Rendering SelectItem
as ComboboxItem
To get the items to function as both a Radix SelectItem
and an Ariakit ComboboxItem
, we have to combine the two components:
<RadixSelect.Item value="en" asChild>
<RadixSelect.ItemText>English</RadixSelect.ItemText>
</RadixSelect.Item>
More examples
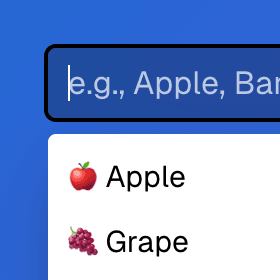
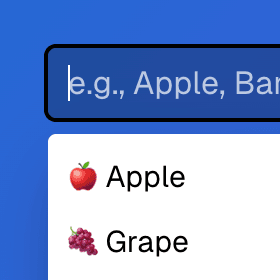
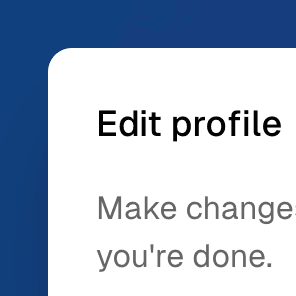
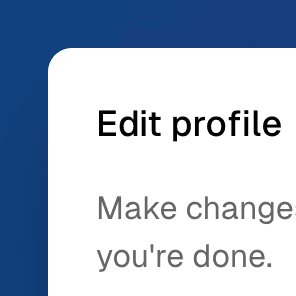
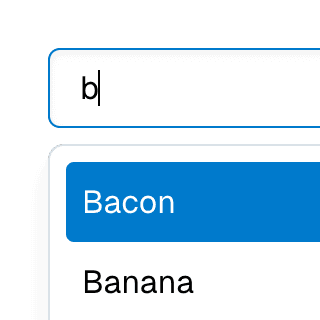
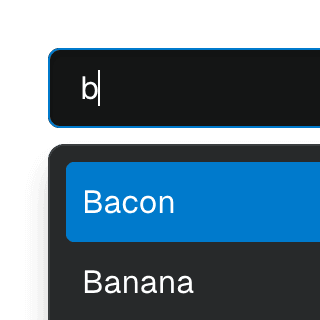
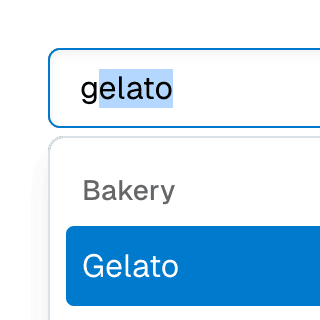
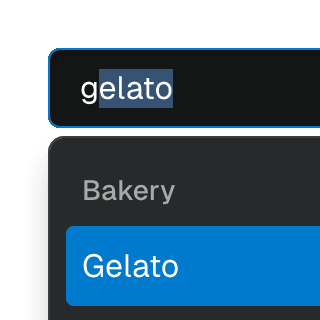
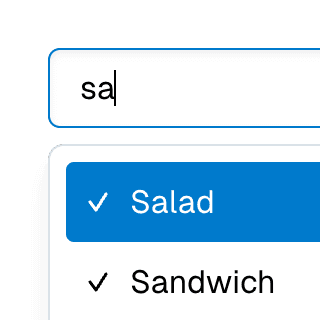
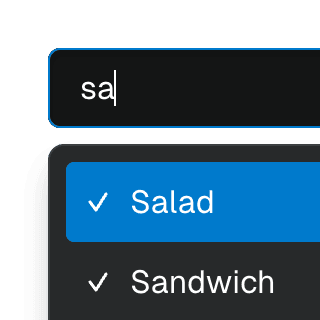
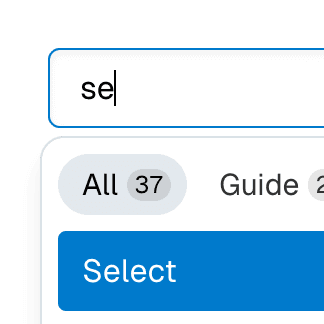
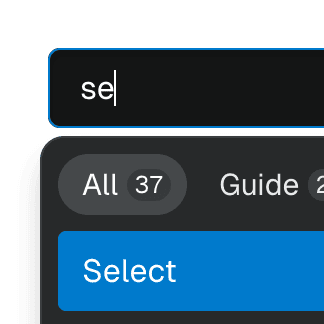