Portal
Renders an element using React Portal.
By default, the portal element is a div
element appended to the
document.body
element. You can customize this with the
portalElement
prop.
The
preserveTabOrder
prop allows this component to manage the tab order of the elements. It
ensures the tab order remains consistent with the original location where the
portal was rendered in the React tree, instead of the final location in the
DOM. The
preserveTabOrderAnchor
prop can specify a different location from which the tab order is preserved.
Code examples
Optional Props
portal
boolean | undefined = true
Determines whether the element should be rendered as a React Portal.
Live examples
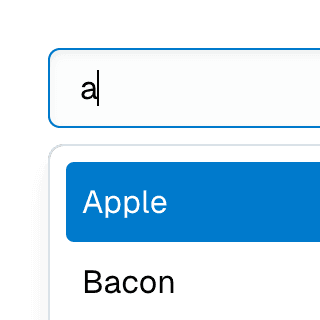
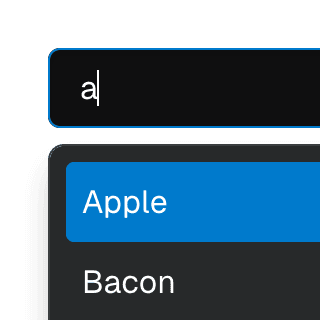
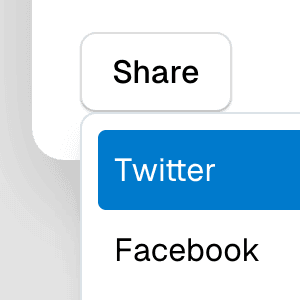
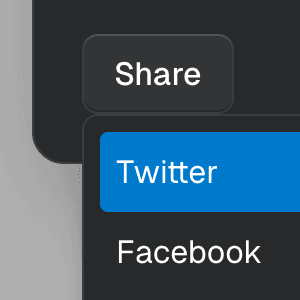
portalElement
HTMLElement | ((element: HTMLElement) => HTMLElement | null) | null | undefined
An HTML element or a memoized callback function that returns an HTML
element to be used as the portal element. By default, the portal element
will be a div
element appended to the document.body
.
Live examples
Code examples
const [portal, setPortal] = useState(null);
<div ref={setPortal} />
const getPortalElement = useCallback(() => {
const div = document.createElement("div");
const portalRoot = document.getElementById("portal-root");
portalRoot.appendChild(div);
return div;
}, []);
portalRef
React.RefCallback<HTMLElement> | React.MutableRefObject<HTMLElement | null> | undefined
portalRef
is similar to ref
but is scoped to the portal node. It's
useful when you need to be informed when the portal element is appended to
the DOM or removed from the DOM.
Live examples
Code examples
preserveTabOrder
boolean | undefined = false
When enabled, preserveTabOrder
will keep the DOM element's tab order the
same as the order in which the underlying
Portal
component was mounted in
the React tree.
If the
preserveTabOrderAnchor
prop is provided, the tab order will be preserved relative to that element.
preserveTabOrderAnchor
Element | null | undefined
An anchor element for maintaining the tab order when
preserveTabOrder
prop is enabled. The tab order will be kept relative to this element.
By default, the tab order is kept relative to the original location in the
React tree where the underlying
Portal
component was mounted.
Code examples
const [anchor, setAnchor] = useState(null);
<button ref={setAnchor}>Order 0</button>
<button>Order 2</button>
// Rendered at the end of the document.
<button>Order 5</button>
// Rendered at the end of the document, but the tab order is preserved.
<button>Order 3</button>
// Rendered at the end of the document, but the tab order is preserved
// relative to the anchor element.
<button>Order 1</button>
<button>Order 4</button>
render
RenderProp<React.HTMLAttributes<any> & { ref?: React.Ref<any> | undefined; }> | React.ReactElement<any, string | React.JSXElementConstructor<any>> | undefined
Allows the component to be rendered as a different HTML element or React component. The value can be a React element or a function that takes in the original component props and gives back a React element with the props merged.
Check out the Composition guide for more details.