FormField
Deprecated:
This component has been renamed to
FormControl
. The API remains
the same.
Abstract component that renders a form field. Unlike
FormInput
, this component
doesn't automatically pass the value
and onChange
props down to the
underlying element. This is so we can use it not only for native form
elements but also for custom components whose value is not controlled by the
native value
and onChange
props.
Code examples
content: "",
},
});
const value = form.useValue(form.names.content);
<Editor
value={value}
onChange={(value) => form.setValue(form.names.content, value)}
/>
}
/>
Required Props
name
StringLike
Field name. This can either be a string corresponding to an existing
property name in the
values
state of
the store, or a reference to a field name from the
names
object in the
store, ensuring type safety.
Live examples
Optional Props
getItem
((props: CollectionStoreItem) => CollectionStoreItem) | undefined
A memoized function that returns props to be passed with the item during its registration in the store.
Code examples
const getItem = useCallback((data) => ({ ...data, custom: true }), []);
id
string | undefined
The unique ID of the item. This will be used to register the item in the
store and for the element's id
attribute. If not provided, a unique ID
will be automatically generated.
Live examples
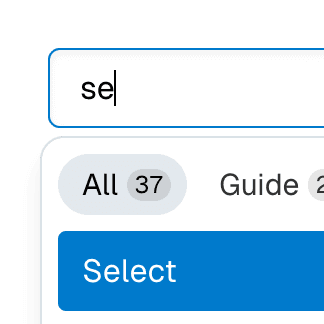
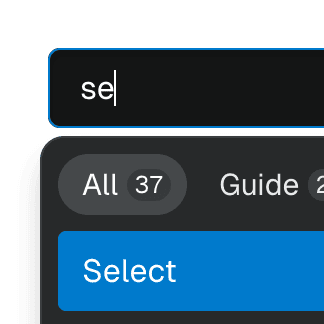
render
RenderProp<React.HTMLAttributes<any> & { ref?: React.Ref<any> | undefined; }> | React.ReactElement<any, string | React.JSXElementConstructor<any>> | undefined
Allows the component to be rendered as a different HTML element or React component. The value can be a React element or a function that takes in the original component props and gives back a React element with the props merged.
Check out the Composition guide for more details.
shouldRegisterItem
boolean | undefined = true
Whether the item should be registered as part of the collection.
store
FormStore<FormStoreValues> | undefined
Object returned by the
useFormStore
hook. If not
provided, the closest Form
or
FormProvider
components'
context will be used.
touchOnBlur
BooleanOrCallback<React.FocusEvent<Element, Element>> | undefined = true
Whether the field should be marked touched on blur.