Dialog
Open a new window that can be either modal
or non-modal and optionally rendered in a React portal
. This component is based on the WAI-ARIA Dialog Pattern.
Examples
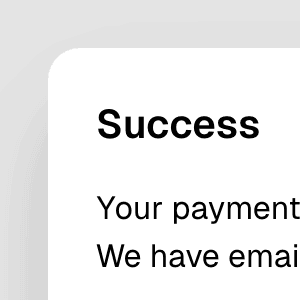
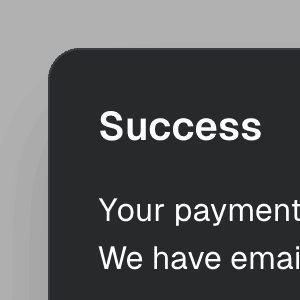
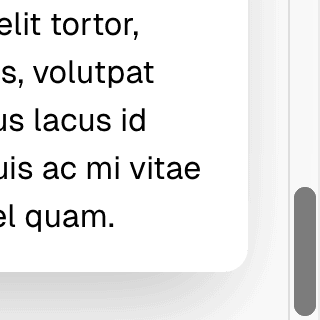
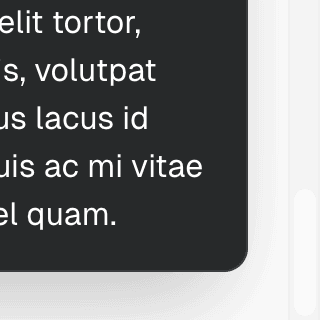
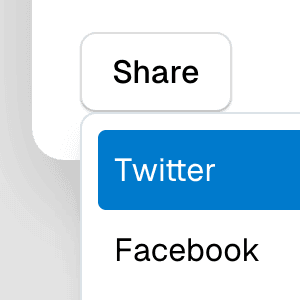
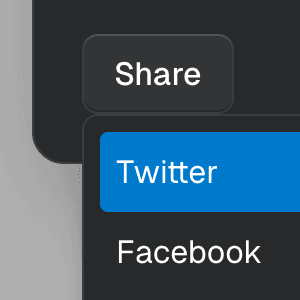
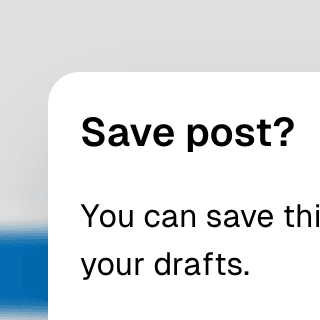
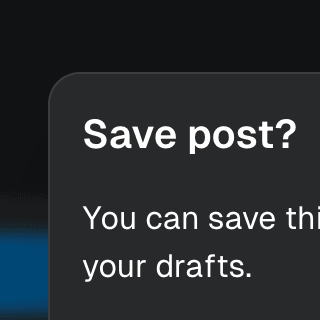
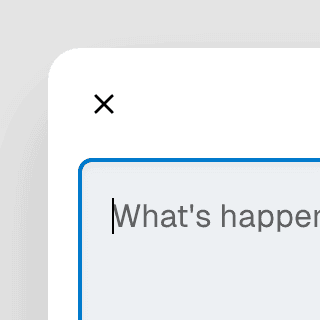
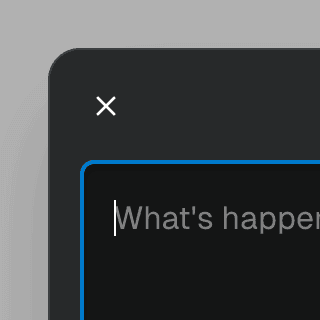
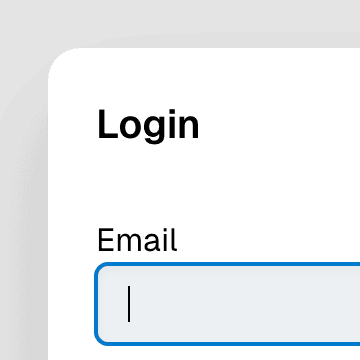
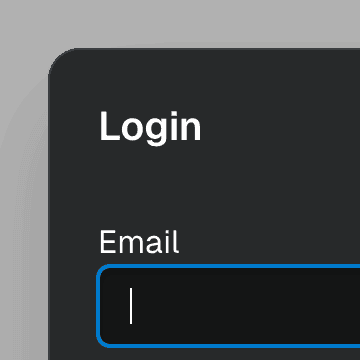
API
Styling
Styling the backdrop
You can style all the backdrop elements using the [data-backdrop]
selector:
[data-backdrop] {
background-color: hsl(0 0 0 / 0.1);
}
To style the backdrop of a specific dialog, use the backdrop
prop:
Scrollbar width
When the preventBodyScroll
prop is set to true
(default for modal
dialogs), the scrollbar will be automatically hidden when the dialog is open. If your page contains position:fixed
elements, you might need to modify their padding to compensate for the missing scrollbar width.
Ariakit automatically defines a --scrollbar-width
CSS variable. You can apply this variable to adjust the padding-right
of your fixed elements:
.header {
padding-right: calc(16px + var(--scrollbar-width, 0));
}
Z-index
Modal dialogs are rendered at the end of the document using React Portal, which means they will be rendered on top of all other elements by default.
However, if you set the portal
prop to false
or use the z-index
property on other elements, you might need to adjust the z-index
of the dialog:
.dialog {
z-index: 100;
}
For more information on styling with Ariakit, refer to the Styling guide.